Go语言笔记
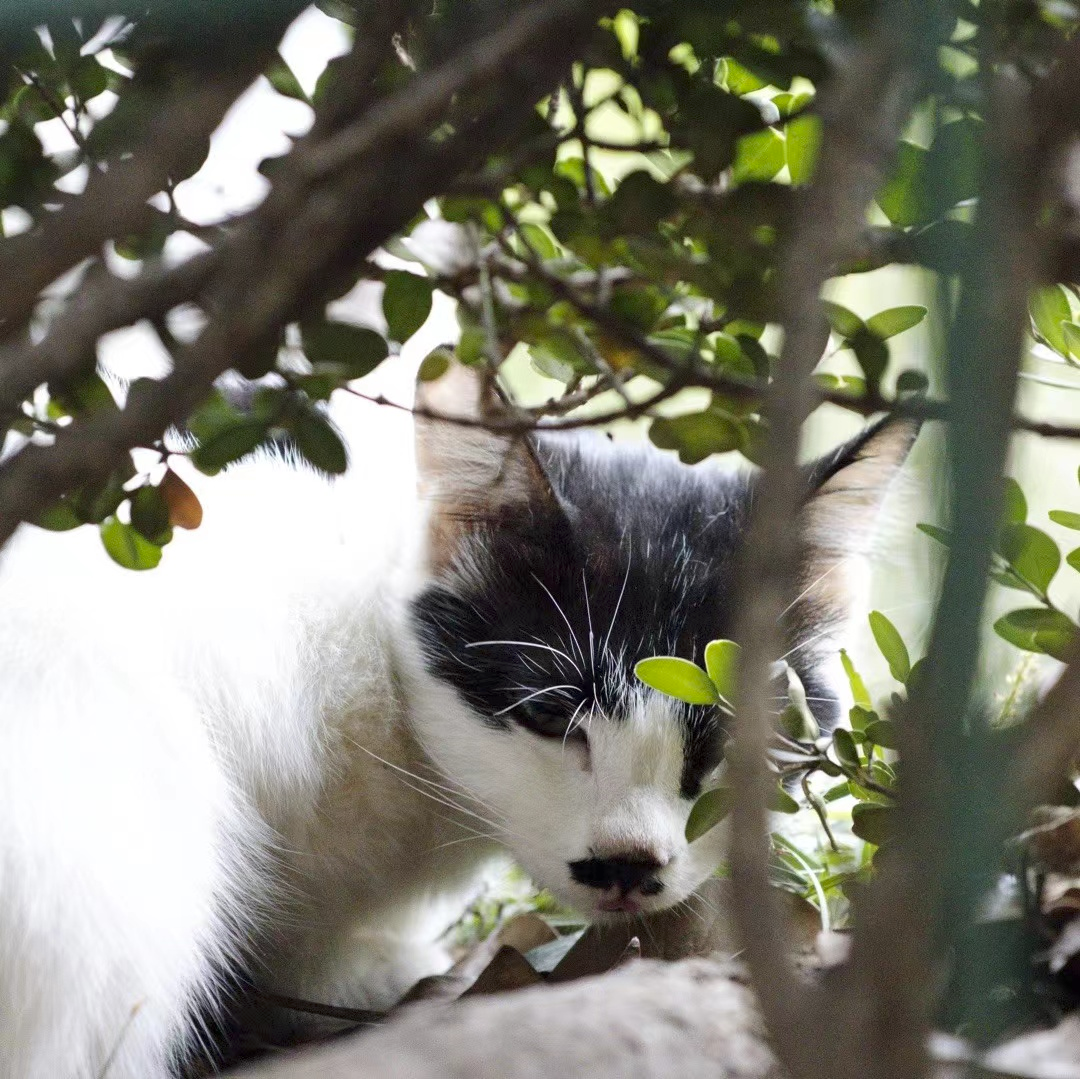
Reference
https://www.runoob.com/go/go-tutorial.html
Dependency
C/C++ programming
Go 程序结构
1 | package main //identify the package, essential, every Go program must start with a package declaration, and the multi-file programs must have main package |
当标识符(包括常量、变量、类型、函数名、结构字段等等)以一个大写字母开头,如:Group1,那么使用这种形式的标识符的对象就可以被外部包的代码所使用(客户端程序需要先导入这个包),(像面向对象语言中的 public);标识符如果以小写字母开头,则对包外是不可见的,但是他们在整个包的内部是可见并且可用的(像面向对象语言中的 protected )。{ 不能放在单独的一行
- 不一定要将文件名与包名定成同一个。
- 文件夹名与包名并非需要一致。
- 同一个文件夹下的文件只能有一个包名,否则编译报错。
Go 基本语法
不压行不需要写';'
- 字符串支持’+’All the blanks above are essential.
Blank Principles 1
2
3
4
5
6
7
8var x int
const Pi float64 = 3.14159265358979323846
if x > 0 {
// do something
}
result := add(2, 3)
1 | import fmt2 "fmt" //alias |
通过
const
关键字来进行常量的定义。通过在函数体外部使用
var
关键字来进行全局变量的声明和赋值。通过
type
关键字来进行结构(struct)和接口(interface)的声明。通过
func
关键字来进行函数的声明。
1 | var b, c int = 1, 2 // multi-assignment is allowed |
- local variable must be used, universal variable can be unused.
Special: iota
1 | package main |
if not given the value, it will automatically inherit the last line.
iota
must be used inconst
, and be set to 1 at eachconst
- operators are the same as C/C++.
- Conditional clause is like C without brackets.
- select clause for channel (NFN)(Not for now)
- Loop is like C, but
for
is the only loop in Go. - Go supports
goto
Go 函数
山东语言(bushi 所有的类型都写在后面
1 | func max(num1, num2 int) int { |
函数可以多值返回,必须接着。
空白标识符 _ 可被用于抛弃值,如值 5 在:_, b = 5, 7 中被抛弃。
_ 实际上是一个只写变量,你不能得到它的值。这样做是因为 Go 语言中你必须使用所有被声明的变量,但有时你并不需要使用从一个函数得到的所有返回值。
- for 语句内可以和外部共用变量名
Go 函数式编程
Go supports closure.
Go ‘OOP’
Go 中实际上没有显式的OOP,但是可以用方法来实现,方法就是给定接收者的函数。因为Go的多文件组织中存在权限机制,所以其实可以按照OOP范式编程。
1 | func (c Circle) getArea() float64 { |
Go array
注意:在 Go 语言中,数组的大小是类型的一部分,因此不同大小的数组是不兼容的,也就是说 [5]int 和 [10]int 是不同的类型。
1 | balance := [...]float32{1000.0, 2.0, 3.4, 7.0, 50.0} |
Go pointer
very similar to C/C++
the empty pointer is nil
(like Scheme)
Go struct
特别的是,使用结构体指针访问结构体成员,不需要使用 ->
操作符,直接使用 .
。
结构体属性也满足前面提到的大小写原则。
- Post title:Go语言笔记
- Post author:Jackcui
- Create time:2023-11-19 21:29:15
- Post link:https://jackcuii.github.io/2023/11/19/go/
- Copyright Notice:All articles in this blog are licensed under BY-NC-SA unless stating additionally.